Top 10 Cybersecurity Practices for Developers
As a developer, your primary concern might be writing clean, efficient code. However, cybersecurity should be high on your list of priorities. Putting a strong defense in place not only protects your users but also safeguards your hard work. Let’s dive into the top 10 cybersecurity practices for developers.
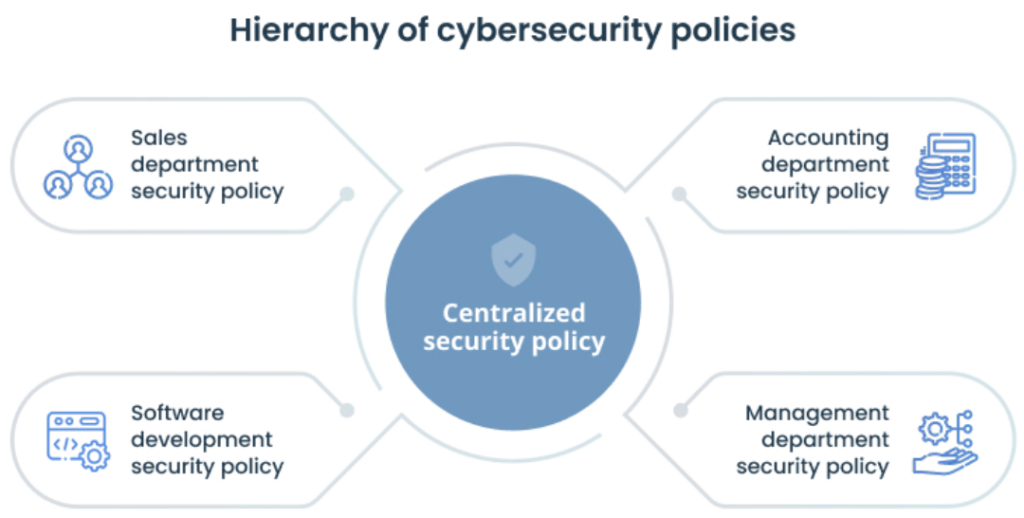
1. Use HTTPS
It should go without saying, but ensuring your website uses HTTPS instead of HTTP is foundational. HTTPS encrypts data transferred between the user’s browser and your servers, protecting sensitive information from interception.
To get started, obtain an SSL certificate from a trusted certificate authority (you can get free certificates from Let’s Encrypt), and then configure your web server to use this certificate.
Example for Apache
ServerName example.com
DocumentRoot /var/www/html
SSLEngine on
SSLCertificateFile /path/to/cert.pem
SSLCertificateKeyFile /path/to/key.pem
2. Sanitize User Input
Gordon Ramsay isn’t the only one who needs to sanitize; as a developer, it’s crucial too! Always validate and sanitize user inputs to fend off threats like SQL injection and XSS attacks.
Example in PHP
$name = htmlspecialchars($_POST['name'], ENT_QUOTES, 'UTF-8');
3. Use Secure Authentication
Password management deserves careful attention. Utilize modern authentication methods, like OAuth, and ensure passwords are securely hashed and salted.
Example Using bcrypt in Node.js
const bcrypt = require('bcrypt');
const saltRounds = 10;
const myPlaintextPassword = 's0/\/\P4$w0rD';
bcrypt.hash(myPlaintextPassword, saltRounds, function(err, hash) {
// Store hash in your password DB.
});
4. Regularly Update and Patch Systems
Patching and updating your systems regularly can prevent a hacker from exploiting known vulnerabilities. Use automated tools to keep track of what needs updating.
For Ubuntu
sudo apt-get update && sudo apt-get upgrade -y
5. Encrypt Sensitive Data
Encrypt sensitive data at rest and in transit. Encryption ensures data is only readable by those possessing the decryption key.
Encrypting Data with OpenSSL
openssl enc -aes-256-cbc -salt -in file.txt -out file.enc
6. Implement Proper Access Controls
Use the principle of least privilege. Ensure users and services only have access to what they need. Implement role-based access control (RBAC) where necessary.
Example in AWS IAM
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"s3:GetObject"
],
"Resource": [
"arn:aws:s3:::example_bucket/*"
]
}
]
}
7. Use Security Headers
Security headers enhance your website’s security. Utilize headers like Content Security Policy (CSP), HTTP Strict Transport Security (HSTS), and X-Frame-Options.
Example for NGINX
add_header Strict-Transport-Security "max-age=31536000; includeSubDomains" always;
add_header X-Frame-Options "DENY";
add_header X-Content-Type-Options "nosniff";
8. Monitor and Log Activities
Logs are your best friend when it comes to tracking unusual activities. Implement comprehensive logging and monitoring to detect any anomalies.
Example Using Node.js and winston
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [
new winston.transports.File({ filename: 'error.log', level: 'error' }),
new winston.transports.File({ filename: 'combined.log' })
]
});
9. Conduct Security Testing
Regularly conduct security testing including code reviews, penetration testing, and vulnerability assessments to identify and mitigate risks.
Using OWASP ZAP
# Run OWASP ZAP in Docker
docker run -u zap -p 8080:8080 owasp/zap2docker-stable zap.sh -daemon -port 8080 -host 0.0.0.0
10. Educate and Train on Security Awareness
Even the best practices won’t help if your team isn’t aware of them. Regularly conduct training sessions to keep your team informed about the latest security threats and best practices.
Conclusion
By adhering to these top 10 cybersecurity practices, you’ll not only be safeguarding your code but also protecting your users. Remember, security isn’t a one-time exercise; it’s an ongoing process.
Are you ready to implement these practices or still stuck in the Stone Age of HTTPS? Don’t leave any stone unturned—your users’ security depends on it!