Secure & Global Cloud Storage
Seamlessly store, share, and sync your files across the globe. No VPN required, even in China.
Why Choose Sesame Disk?
Experience secure, global, and fast cloud storage with unmatched features.
π Global Sync
Access your files from anywhere, even in restricted regions.
π‘ Secure Storage
Your files are encrypted and safely stored in our cloud.
β‘ Fast File Transfers
Optimized servers ensure high-speed uploads and downloads.
π Seamless Collaboration
Office and project files managed effortlessly.
π Snapshot Backups
Recover files with up to 2 years of snapshots.
π Developer API
Integrate with your own apps using our REST API.
End-to-End Encryption
Your data is protected with the highest security standards.
Flexible Pricing
Choose a plan that fits your needs without hidden costs.
Get Started for Free
Enjoy secure, high-speed cloud storage with global accessβno credit card required.
Explore Our Features
Discover how our cloud services can enhance your workflow. Watch these videos to learn more about our multi-user plans and seamless folder synchronization with other cloud providers.
Multi-user Plans Features
Here you have a short video showcasing some of the features available in our multi-user plans.
Sync Local Folders with Cloud
Learn how to keep your local folders updated with Dropbox, Google Cloud, Microsoft OneDrive, and more.
Plans and Pricing
All plans include a set amount of storage & traffic in the price, but you can expand them on demand.
π Get 10% off with an annual subscription! π
Free Plan
$0/month
- π¦ 2GB Storage
- π€ Single User
- π End-to-end encryption
- π Secure file sharing
- π± Multi-device sync
250GB Starter
$4/month
- π End-to-end encryption
- π Secure file sharing
- π± Multi-device sync
- β‘ High-speed transfers
- π₯ Multi-user support
2TB StarterPlus
$10/month
- π End-to-end encryption
- π Secure file sharing
- π± Multi-device sync
- β‘ High-speed transfers
- π₯ Multi-user support
8TB Business
$40/month
- π End-to-end encryption
- π Secure file sharing
- π± Multi-device sync
- β‘ High-speed transfers
- π₯ Multi-user support
π° 30-day money-back guarantee β try Sesame Disk risk-free!
πΌ Bulk discounts available for teams! Contact us for special pricing.
What Our Customers Say
Francis Lim
Ops
"Most cloud services struggle in China. NiHao Cloud solved the problem. It really works!"
Lea Chateau
Business Owner
"We send files from Canada to China. NiHao Cloud is cost-effective and reliable!"
Karen Baartmans
Cloud User
"Great format and features. If you need cloud file transfers, this is the solution!"
Christoph Kendler
CEO
"Before using NiHao Cloud, slow connections disrupted our work. Now everything flows smoothly."
Michael Dowson
Film Director
"We transfer large video files to China. NiHao Cloud is fast and secure. Highly recommended!"
Maria J. Mathews
Director
"Our global team struggled with file sharing. NiHao Cloud changed everything! Their customer support is amazing."
Frequently Asked Questions
How secure is my data?
We use end-to-end encryption, ensuring only you have access to your files.
Can I add more users to my plan?
Yes! Our plans support multiple users, with volume discounts available.
Is there a free trial?
Yes! We offer a free trial so you can explore Sesame Disk risk-free.
Do you offer a money-back guarantee?
Yes! Here at Sesame Disk Group, we believe our cloud will transform the way you share files. Weβre so sure of this that we offer a 30-day money-back guarantee. If you are not happy with our services or donβt feel it is for you, simply contact us to get the entire cost of the first month refunded.
Is your Cloud Service available in the Chinese Mainland?
Yes, our Cloud Storage service is available in China and works without needing a VPN. The software also includes Chinese language support. Although some domains may be blocked, we have alternative domains and IPs to ensure accessibility.
Can I use Sesame Disk by NiHao Cloud in the United States?
Yes! Our servers are located worldwide, making Sesame Disk accessible globally, including the USA, EU, and Africa.
Does your Storage Cloud provide the same service as Google Drive or Dropbox?
Yes, NiHao Cloud offers services similar to Dropbox, Google Drive, and Box. However, our niche is cross-border file sharing and team collaboration, especially for markets like Mainland China.
Does your service share files with the Chinese government?
Absolutely not. At Sesame Disk / NiHao Cloud, we prioritize your privacy. Our servers are not located in China, and even our engineers cannot access your data.
Is WeTransfer, Dropbox, Google Drive & OneDrive blocked in Mainland China?
Yes, these services are not accessible in China without a VPN. Sesame Disk by NiHao Cloud offers an alternative solution for users in China without requiring a VPN.
What is the best way to send files to Mainland China?
The best way to send files to Mainland China is by using a service like Sesame Disk by NiHao Cloud. Our platform allows seamless file sharing without VPN restrictions.
Contact Us
Have questions? Get in touch with us.
Need quick assistance? Visit our Help Center for FAQs and guides.
You can also receive immediate assistance by clicking the chat icon in the bottom right corner to talk with our specialized chatbot.
About Us
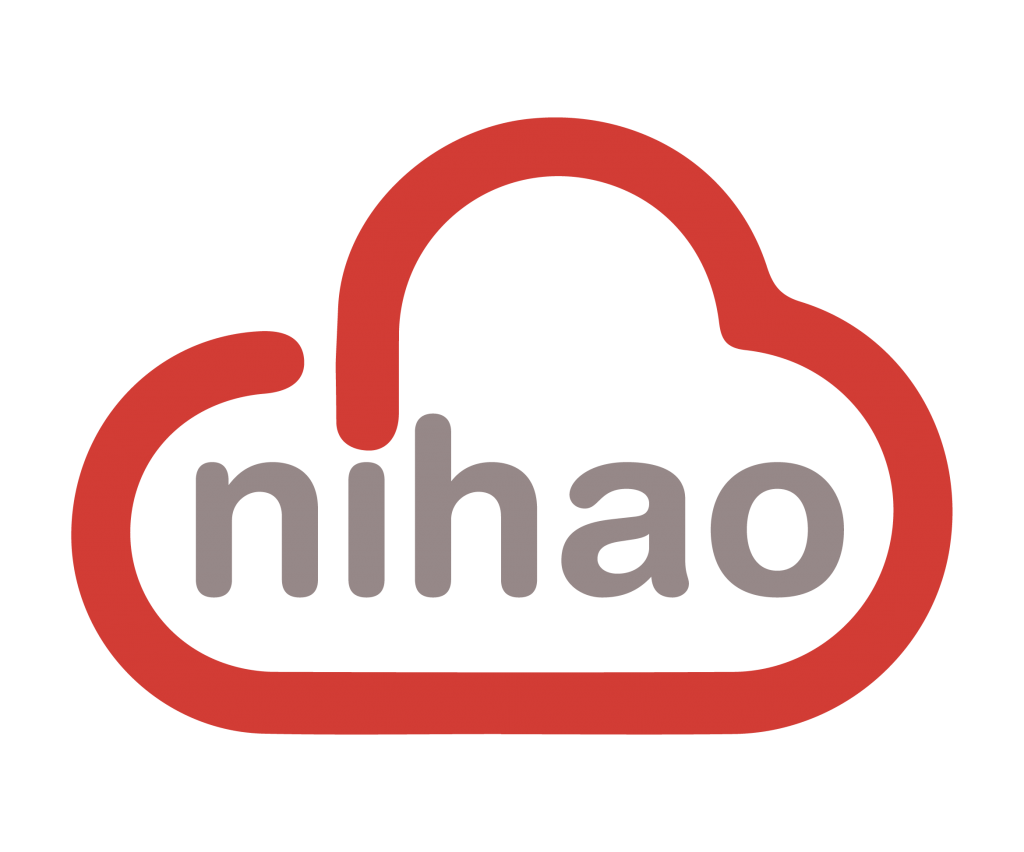
Sesame Disk by NiHao Cloud was founded by a distributed team across Mainland China, APAC, Australia, Europe, Africa, and the USA. Facing challenges in safe file-sharing and collaboration, we built a solution that ensures secure, fast, and seamless data exchange worldwide.
Our Mission
We empower individuals and businesses to collaborate effortlessly across borders. Whether itβs sharing large files, securing sensitive data, or working in real-time, we provide a reliable cloud infrastructure that optimizes performance worldwide.
Global Accessibility
Our optimized data routes ensure stable and fast access to files, even in regions like Mainland China, without requiring VPNs. We serve businesses globally, ensuring seamless collaboration across all industries.
Explore Our Roadmap
We are constantly innovating and improving our services. Check out our roadmap to see upcoming features, improvements, and how we are shaping the future of cross-border cloud storage.
View Our RoadmapOur Journey
Originally launched as an internal IT project within IBB I&C, a German IT provider, our cloud solution quickly evolved. In 2016, we expanded as IBB Cloud and, by 2017, rebranded to NiHao Cloud. In 2020, we introduced Sesame Disk, enhancing cross-border cloud storage efficiency worldwide.