Cracking the Code: Exploring Recursion in GoLang – Functions Calling Themselves
If you’re looking for a post that gets into the thick and thin of GoLang, then you’re in the right place! To prevent anyone from getting stuck in an infinite loop, our discussion today will focus on recursion in GoLang where functions call themselves.
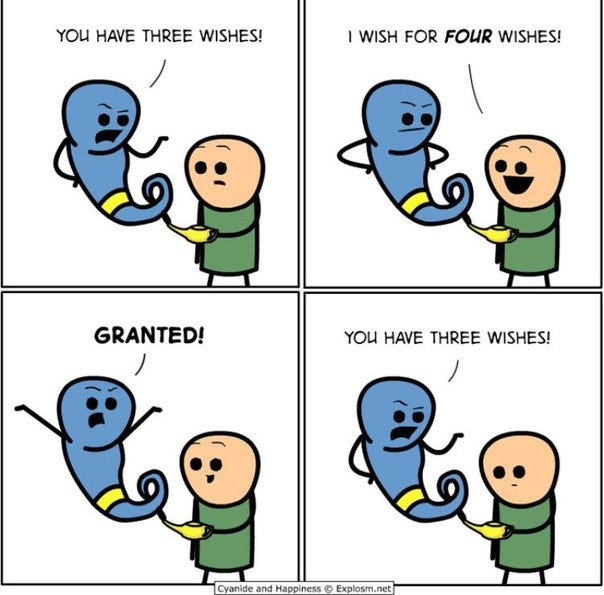
Lost? Don’t worry. In computer science, recursion is nothing more than a method where the solution to a problem is based on solving smaller instances of the same problem. It’s like Russian nesting dolls – but in code.
About GoLang
Before diving into the beauty of recursion, we need to understand the beast we’re dealing with – GoLang. Launched in 2007 by Google, Go is a statically typed, compiled language that boasts simplicity and efficiency. If it is daunting enough, put your worries at bay, because it’s designed to be easy to understand and write. For a comprehensive guide to GoLang, check Effective Go.
Understanding Recursion
If you’ve made it this far, it’s clear that you’re not one to back down from a challenge. Come on, let’s level-up your GoLang game with recursion.
A simple way to look at recursion is a function that calls itself until it doesn’t. Well, that’s a circular definition if I’ve ever heard one! Sort of like saying recursive functions are like shampoo instructions – “Lather, Rinse, Repeat”.
Now, let’s look at an example. Consider calculating the factorial of a number. The traditional iterative approach comes naturally to us:
func factorial(n int) int {
result := 1
for ; n > 0; n-- {
result *= n
}
return result
}
It works, but here’s how recursion can achieve the same result:
func factorial(n int) int {
if n == 0 {
return 1
}
return n * factorial(n-1)
}
This recursive function keeps calling itself, reducing the problem size step by step, until it becomes simple enough to be solved directly i.e., the base case.
When to Use Recursion in GoLang
“Can I use recursion all the time then?”, you might ask. Well, like most things in life, it depends. Using recursion might make your code cleaner and easier to understand. However, recursive functions could also become very inefficient if not used properly. Therefore, pick your cases wisely.
Debugging Recursion in GoLang
If you’re caught in a recursive loop, don’t panic! Debugging recursion can be done with some old fashioned print statements. For instance:
func factorial(n int) int {
fmt.Println("factorial", n)
if n == 0 {
return 1
}
result := n * factorial(n-1)
fmt.Println("return", result)
return result
}
This will give you a step-by-step trace of your program’s execution.
Information is pretty fluid across the technological sphere. The more you expose yourself and acquire it, the better equipped you become in crafting optimized solutions. Recursion is a handy tool in your developer kit, so tinker around with it. After all, practice doesn’t make perfect, perfect practice does!
“With recursion, you can write compact and elegant programs that fail spectacularly at runtime.” – Unknown.
So, go ahead! Master recursion and revolutionize your GoLang coding journey. Because why go for the ordinary when you can GO for the extraordinary?
To finish off, if you think you’ve understood recursion, go back to the beginning of this post and read it again. Just kidding! Or am I?